API Documentation (Using R)
Username: This is the email address associated with your TruMedia account.
Sitename: This is the name of your site. This is typically the first part of the URL of your site (ex: sitename of rangers.trumedianetworks.com is rangers).
Temporary Token:
Temporary authentication tokens allow a URL to be authorized and accessed without login. In order to create a temporary token, a master token is required. The master token should remain private. Only the temporary token is exposed in the URL. Temporary authentication tokens will last for 24 hours by default, but can be requested to last for any duration up to 72 hours.
The two primary use cases for temporary authentication tokens are:
To implement an SSO integration allowing users of your internal site to access the TruMedia site without being required to log in separately.
To access the TruMedia Data and Image API’s
Master Token:
These are currently provided by TruMedia. In a future release, they will be generated and managed within the TruMedia application by team Super Users. Using your Username, Sitename, and Master Token, you can use the code below to create a Temporary Token:
Code Template - Paste In Your Username, Sitename, and Master Token (for R):
#Downloading packages
require(curl)
require(httr)
require(dplyr)
#Generating token
headers <- c('Content-Type' = 'application/json')
data <- '{"username":"user@email.com", "sitename":"sitename", "token":"master token"}'
res <- httr::POST(url = 'https://api.trumedianetworks.com/v1/siteadmin/api/createTempPBToken', httr::add_headers(.headers=headers), body = data)
token <- content(res)
tok <- token$pbTempToken
Pulling Data
A user can pull data by editing parts of the query URL. Below is the URL:
data <- read.csv(paste(“https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/dataFormat.csv?”, “param1”, param1, “¶m2=”, param2, “&columns=”, columns, filters, qualifications, sort, “&token=”, tok, sep = ““)
Adjusting Data Format
This will adjust the format that the data is presented, changing what each row represents, The options for dataFormat are TeamTotals, TeamSeasons, TeamGames, TeamPlays, PlayerTotals, PlayerSeasons, PlayerGames, PlayerPlays, and GamePitches. You can also change the file type to JSON or CSV.
Adding Parameters
This can narrow the dataset to only what you want to see. In order to add parameters, you will need to declare the parameter and its settings before the query URL. In the query URL, you will need to add the parameter and its contents into the URL. To see all available parameters, please see the list below:
seasonYear: Satisfies requirement for all team queries and player queries.
For single years:
seasonYear <- 2023
For multiple years:
seasonYear <- “2021%2C2022%2C2023”
You can also remove this from the query and use season filters in the Adding Filters section
seasonLevel: Satisfies requirement for Team Totals and Team Seasons queries. Level of play (MLB, AAA, AAX, AFA, AFX, ASX, ROK, AFL, MEX, WIN, NPB, KBO)
gameType: Comma delimited list of game types (REG, WC, DS, LCS, WS, PRE)
gameId: Satisfies requirement for all queries. Comma delimited list of MLB Game PK values. Max of 100 game ID’s for team queries, 25 for player queries.
gameString: Satisfies requirement for all queries. Comma delimited list of MLB Game String values (format: gid_YYYY_MM_DD_HOMETM_AWAYTM_N). Max of 100 game strings for team queries, 25 for player queries.
teamId: Comma delimited list of up to 10 MLB Team ID’s (except for Team Plays query which takes only 1 team ID). Satisfies requirement for all team queries as well as the Player Totals and Players Seasons queries.
orgId: MLB Org ID. Satisfies requirement for Team Totals and Team Seasons queries.
opponentId: Comma delimited list of up to 10 MLB Team ID’s
playerId: Comma delimited list of up to 50 MLB Player ID’s (except for Player Plays query which takes only 1 player ID). Satisfies requirement for all player queries.
statEvent: Required for the Team Plays and Player Plays query, this defines the stat for events being queried. More information on this parameter can be found in the “TruMedia Data Service Filter Querying” document.
Format: There are three options for this parameter:
FORMATTED - formatted value (ex: ‘.123’, ‘12%’, etc)
RAW - raw decimal values
MIXED - For JSON only, an array with both the formatted and raw values.
Note: For every additional parameter, you will need to include an ampersand (“&”) when listing them in the query URL.
Example: “seasonYear”, seasonYear, “&seasonType=”, seasonType, “&week=”, week,…
Adding Columns
Using the native stat abbreviations, you can list the columns you want to pull in this format:
columns <- “[stat1],[stat2]”
To see all available stats, please visit your site’s glossary.
Note: Make sure to use brackets around stat abbreviations and do not leave any spaces in column listing.
Example:
columns <- “[1B],[HR],[SLG]”
Adding Filters
To add filters to your query URL, you can use this helpful tool by visiting this tool. Put your site URL prefix into the red italicized section below: https://insert_your_site_url_here.trumedianetworks.com/baseball/tools?pd=%7B"activeTab"%3A"queryTool"%7D
Once one this page, click on the ‘Search Filters’ dropdown and search for the desired filters. Once complete, click on the ‘Show Where Clause’ button and copy the text from the second section into your filter declaration statement
Example:
filters <- “&filters=((event.outs%20IN%20('2')))”)
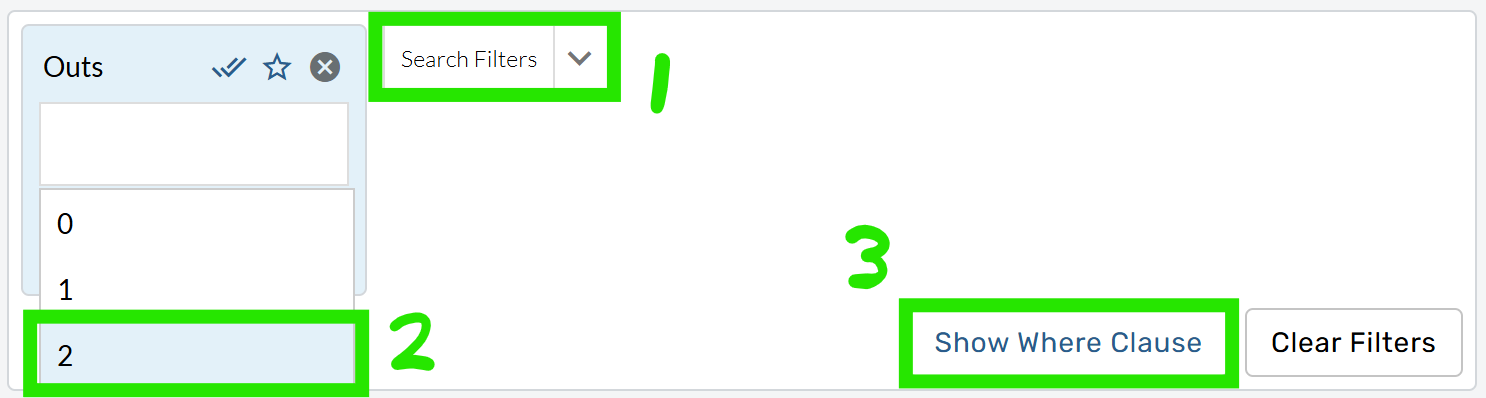
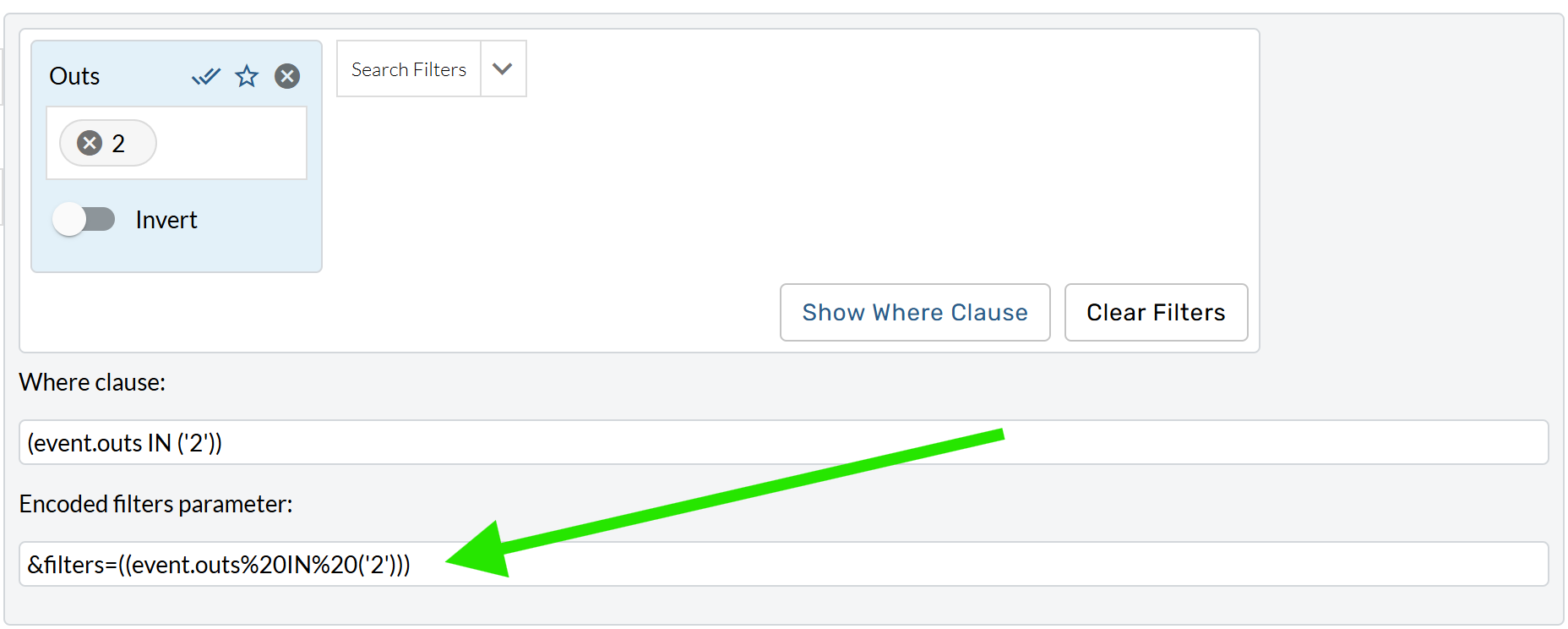
Adding Qualifications
Optional qualification clause for limiting results. Applies to all queries except Plays queries. This parameter is essentially like using the Qualifications UI control on a player or team page. The URL string is:
qualifications <- “qualification=%5Bstat1%5D+%3D+number”
For example, if you wanted to set a qualification for the query results to only include players with 100 or more at-bats, you would use the following as your qualifications declaration statement (printed before the query):
qualifications <- “qualification=%5BAB%5D+%3D+100”
Note: Qualifications will be applied before filters in the query statement. For example, if a user builds a query to find batting statistics among players with 100+ at-bats, the 100+ at-bat qualification will be implemented first (narrowing the query to players with 500+ total attempts) and the batting statitics with the applied filters will follow.
Adding Sort
Optional order clause for sorting the results. This parameter is essentially like clicking on a stat column header in the UI to sort the report by that stat. The URL string is:
sort <- “&sort=%5Bstat1%5D+ORDER”
For example, if you wanted to sort the query results by at-bats descending, you would use the following as your qualifications declaration statement (printed before the query):
sort <- “&sort=%5BAB%5D+DESC”
If the stat you wanted to sort the query results by includes a percent sign (example: “BB%”), you can use the follow statement (adding “25” after the percent sign):
sort <- "&sort=%5BBB%25%5D+DESC"
Data Query Examples
Below is an example pulling homeruns by team totals in the seventh inning or after in the 2024 regular season in descending order:
#Authentification
##Copy and paste code from the Getting Started section
#Setting parameters for the 2024 MLB Regular Season
seasonYear <- 2024
seasonLevel <- "MLB"
gameType <- "REG"
statEvent <- "[G]"
#Setting the columns for homeruns
columns <- "[HR]"
#Adding filter for the 7th inning or after (copied from results from filter URL generation process)
filters <- "&filters=((event.inning%20%3E%3D%207))"
#Adding sort for homeruns descending
sort <- "&sort=%5BHR%5D+DESC"
#Query Statement
data <- read.csv(paste("https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/TeamTotals.csv?","seasonYear=", seasonYear, "&seasonLevel=", seasonLevel, "&gameType=", gameType,"&columns=", columns,"&statEvent=",statEvent, filters, sort,"&token=", tok, sep = ""))
head(data, 5)
Below is an example of pulling pitching stats from player totals in the since 2015 (regular season and playoffs) that were on the Texas Rangers and playing at Yankee Stadium with at least five innings pitched sorted by ERA ascending:
#Authentification
##Copy and paste code from the Getting Started section
#Setting parameters for Regular Season & Playoff Games since 2015
seasonYear <- “2015%2C2016%2C2017%2C2018%2C2029%2C2020%2C2021%2C2022%2C2023%2C2024”
seasonLevel <- "MLB"
gameType <- "REG,WC,DS,LCS,WS"
statEvent <- "[G]"
#Setting the columns
columns <- "[G],[IP],[ERA],[AVG|PIT],[OPS|PIT]"
#Adding filter for Yankee Stadium, adding Team ID for Texas Rangers
filters <- "&filters=((game.gameVenueId%20IN%20('3313')))"
team <- 140
#Adding sort for ERA descending
sort <- "&sort=%5BERA%5D+ASC"
#Adding qualification for at least five innings pitched
qualifications <- "&qualification=%5BIP%5D+%3E%3D+5"
#Query Statement
data <- read.csv(paste("https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/PlayerTotals.csv?","seasonYear=",seasonYear,"&seasonLevel=", seasonLevel,"&gameType=", gameType,"&team=", team,"&columns=", columns, qualifications, "&statEvent=",statEvent, filters, sort,"&token=", tok, sep = ""))
head(data, 5)
Below is an example of pulling hitting stats from player totals in the 2024 Regular Season that were in the AL West with at least 100 games played sorted by BA descending:
#Authentification
##Copy and paste code from the Getting Started section
#Setting parameters for the 2024 MLB Regular Season
seasonYear <- 2024
seasonLevel <- "MLB"
gameType <- "REG"
statEvent <- "[G]"
#Setting the columns for hitting stats
columns <- "[G],[BA],[HR],[RBI],[OPS]"
#Adding sort for batting average descending
sort <- "&sort=%5BBA%5D+DESC"
#Adding qualification for at least 100 games played
qualifications <- "&qualification=%5BG%5D+%3E%3D+100"
#Adding filter AL West (copied from results from filter URL generation process)
filters <- "&filters=((team.game.gameDivision%20IN%20('AL%20West')))"
#Query Statement
data <- read.csv(paste("https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/PlayerTotals.csv?","seasonYear=",seasonYear,"&seasonLevel=", seasonLevel,"&gameType=", gameType,"&columns=", columns, qualifications, "&statEvent=",statEvent, filters, sort,"&token=", tok, sep = ""))
head(data, 5)
Below is an example of pulling hitting stats from player totals in the 2024 Regular Season & Playoffs that were on the Texas Rangers against left handed pitchers with at least 100 plate appearances sorted by walk percentage descending:
#Authentification
##Copy and paste code from the Getting Started section
#Setting parameters for the 2024 MLB Regular Season and Playoffs
seasonYear <- 2024
seasonLevel <- "MLB"
gameType <- "REG,WC,DS,LCS,WS"
statEvent <- "[PA]"
#Setting the columns
columns <- "[G],[PA],[BA],[HR],[RBI],[SLG],[OPS],[BB%]"
#Adding filter for Left Handed Pitchers, adding Team ID for Texas Rangers
filters <- "&filters=(((event.pitcherHand%20%3D%20'L')))"
team <- 140
#Adding sort for Walk% descending
sort <- "&sort=%5BBB%25%5D+DESC"
#Adding qualification for at least 100 plate appearances
qualifications <- "&qualification=%5BPA%5D+%3E%3D+100"
#Query Statement
data <- read.csv(paste("https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/PlayerTotals.csv?","seasonYear=",seasonYear,
"&seasonLevel=", seasonLevel,"&gameType=", gameType,"&team=", team,"&columns=", columns, qualifications, "&statEvent=",statEvent, filters, sort,"&token=", tok, sep = ""))
head(data, 5)
Below is an example of pulling baserunning stats from player totals in the 2024 Regular Season & Playoffs that with at least 10 stolen base attempts sorted by stolen bases descending:
#Authentification
##Copy and paste code from the Getting Started section
#Setting parameters for the 2024 MLB Regular Season and Playoffs
seasonYear <- 2024
seasonLevel <- "MLB"
gameType <- "REG,WC,DS,LCS,WS"
statEvent <- "[SBA]"
#Setting the for baserunning columns
columns <- "[G],[SBA],[SB],[CS],[SBA2],[CS2],[SB2%],[CS%]"
#Adding sort for stolen bases descending
sort <- "&sort=%5BSB%5D+DESC"
#Adding qualification for at least 10 stolen base attempts
qualifications <- "&qualification=%5BSBA%5D+%3E%3D+10"
#Query Statement
data <- read.csv(paste("https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/PlayerTotals.csv?","seasonYear=",seasonYear,
"&seasonLevel=", seasonLevel,"&gameType=", gameType,"&team=","&columns=", columns, qualifications, "&statEvent=",statEvent, filters, sort,"&token=", tok, sep = ""))
head(data, 5)
Below is an example pulling homeruns by team totals in one run games in the 2024 regular season in win percentage descending order:
#Authentification
##Copy and paste code from the Getting Started section
#Setting parameters for the 2024 MLB Regular Season
seasonYear <- 2024
seasonLevel <- "MLB"
gameType <- "REG"
statEvent <- "[G]"
#Setting the columns for win/loss
columns <- "[Win%],[W],[L]"
#Adding filter for one run games (copied from results from filter URL generation process)
filters <- "&filters=((team.game.totalRunDiff%20%3E%3D%20-1)%20AND%20(team.game.totalRunDiff%20%3C%3D%201))"
#Adding sort for win% descending
sort <- "&sort=%5BWin%25%5D+DESC"
#Query Statement
data <- read.csv(paste("https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/TeamTotals.csv?","seasonYear=", seasonYear, "&seasonLevel=", seasonLevel, "&gameType=", gameType,"&columns=", columns,"&statEvent=",statEvent, filters, sort,"&token=", tok, sep = ""))
head(data, 5)
Below is an example of pulling hitting stats from player games in the 2024 Regular Season that were at Wrigley Field by Dansby Swanson:
#Authentification
##Copy and paste code from the Getting Started section
#Setting parameters for the 2024 MLB Regular Season
seasonYear <- 2024
seasonLevel <- "MLB"
gameType <- "REG"
#Dansy Swanson PlayerId
playerId <- 621020
#Setting the columns for hitting statistics
columns <- "[G],[BA],[HR],[RBI],[OPS]"
#Adding filter for one run games (copied from results from filter URL generation process)
filters <- "&filters=((game.gameVenueId%20IN%20('17')))"
#Query Statement
data <- read.csv(paste("https://api.trumedianetworks.com/v1/mlbapi/custom/baseball/DirectedQuery/PlayerGames.csv?","seasonYear=", seasonYear, "&seasonLevel=", seasonLevel, "&gameType=", gameType,"&playerId=",playerId, "&columns=", columns, filters,"&token=", tok, sep = ""))
head(data, 5)